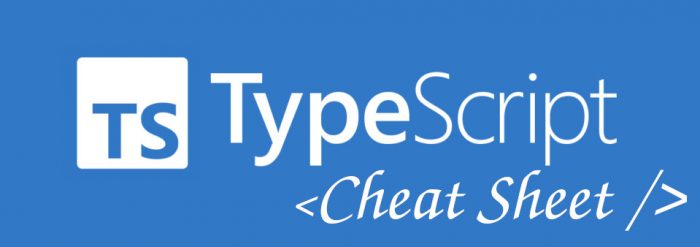
Guía de referencia rápida de TypeScript 4.3
Operadores | |
?? Fusión nula |
function getValue(val?: number): number | 'nil' { // Devolverá 'nil' si 'val' es falso (incluido 0) // retorna val || 'nil'; // Solo devolverá 'nil' si 'val' es null o undefined return val ?? 'nil'; } |
?. Encadenamiento opcional |
function countCaps(value?: string) { // La expresión 'value' no estará definida si 'value' es null o // undefined, o si la llamada 'match' no encuentra nada return value?.match(/[A-Z]/g)?.length ?? 0; } |
! (afirmación nula) |
let value: string | undefined; // Código que estamos seguros que se inicializará y 'value' // no se será undefined // Afirmamos que 'value' está definido console.log(`value tiene ${value!.length} caracteres de largo`); |
&&= Operado asignación lógica AND |
let a; let b = 1; // asignar un valor solo si el valor actual es verdadero a &&= 'default'; // a es undefined b &&= 5; // b ahora es 5 |
||= Operador de asignación lógica OR |
let a; let b = 1; // asigna un valor solo si el valor actual es falso a ||= 'default'; // a ahora es 'default' b ||= 5; // b es todavia 1 |
??= Operador de asignación nula |
let a; let b = 0; // asignar un valor solo si el valor actual es null o undefined a ??= 'default'; // a es ahora 'default' b ??= 5; // b sigue siendo 0 |
Tipos básicos | |
Sin tipo |
any |
Una cadena |
string |
Un valor verdadero / falso |
boolean |
Un valor no primitivo |
object |
Valor no inicializado |
undefined |
Valor explícitamente vacío |
null |
Nulo o indefinido (generalmente solo se usa para retornos de funciones) |
void |
Un valor que nunca puede ocurrir |
never |
Un valor con un tipo desconocido |
unknown |
Tipos de objetos | |
Object |
{ requiredStringVal: string; optionalNum?: number; readonly readOnlyBool: bool; } |
Objeto con propiedades de cadena arbitrarias (como un mapa hash o un diccionario) |
{ [key: string]: Type; } { [key: number]: Type; } |
Tipos literales | |
String |
let direction: 'left' | 'right'; |
Number |
let roll: 1 | 2 | 3 | 4 | 5 | 6; |
Arrays y tuplas | |
Array de strings |
string[] // o Array |
Array de funciones que devuelven strings |
(() => string)[] // o { (): string; }[] // o Array<() => string> |
Tuplas básicas |
// opcion 1 let myTuple: [ string, number, boolean? ]; // opcion 2 myTuple = [ 'test', 42 ]; |
Tuplas variádicas |
type Numbers = [number, number]; type Strings = [string, string]; type NumbersAndStrings = [...Numbers, ...Strings]; // [number, number, string, string] type NumberAndRest = [number, ...string[]]; // [number, variable number de string] type RestAndBoolean = [...any[], boolean]; // [variable number de any, boolean] |
Tuplas con nombre |
type Vector2D = [x: number, y: number]; function createVector2d(...args: Vector2D) {} // function createVector2d(x: number, y: number): void |
Funciones | |
Función |
(arg1: Type, argN: Type) => Type; // o { (arg1: Type, argN: Type): Type; } |
Constructor |
new () => ConstructedType; // o { new (): ConstructedType; } |
Función con parámetro opcional |
(arg1: Type, optional?: Type) => ReturnType |
Función con parámetro de rest |
(arg1: Type, ...allOtherArgs: Type[]) => ReturnType |
Función con propiedad estática |
{ (): Type; staticProp: Type; } |
Función con argumento por defecto |
function fn(arg1 = 'default'): ReturnType {} |
Función flecha |
(arg1: Type): ReturnType => { ...; return value; } // o (arg1: Type): ReturnType => value; |
Tipo this |
function fn(this: Foo, arg1: string) {} |
Sobrecarga de funciones |
function conv(a: string): number; function conv(a: number): string; function conv(a: string | number): string | number { ... } |
Tipos de unión e intersección | |
Unión |
let myUnionVariable: number | string; |
Intersección |
let myIntersectionType: Foo & Bar; |
Tipos nombrados | |
Interface |
interface Child extends Parent, SomeClass { property: Type; optionalProp?: Type; optionalMethod?(arg1: Type): ReturnType;} |
Clase |
class Child extends Parent implements Child, OtherChild { property: Type; defaultProperty = 'default value'; private _privateProperty: Type; private readonly _privateReadonlyProperty: Type; static staticProperty: Type; constructor(arg1: Type) { super(arg1); } private _privateMethod(): Type {} methodProperty: (arg1: Type) => ReturnType; overloadedMethod(arg1: Type): ReturnType; overloadedMethod(arg1: OtherType): ReturnType; overloadedMethod(arg1: CommonT): CommonReturnT {} static staticMethod(): ReturnType {} subclassedMethod(arg1: Type): ReturnType { super.subclassedMethod(arg1); } } |
Enum |
enum Options { FIRST, EXPLICIT = 1, BOOLEAN = Options.FIRST | Options.EXPLICIT, COMPUTED = getValue() } enum Colors { Red = "#FF0000", Green = "#00FF00", Blue = "#0000FF" } |
Type alias |
type Name = string; type Direction = 'left' | 'right'; type ElementCreator = (type: string) => Element; type Point = { x: number, y: number }; type Point3D = Point & { z: number }; type PointProp = keyof Point; // 'x' | 'y' const point: Point = { x: 1, y: 2 }; type PtValProp = keyof typeof point; // 'x' | 'y' |
Genéricos | |
Función usando parámetros de tipo |
(items: T[], callback: (item: T) => T): T[] |
Interfaz con múltiples tipos |
interface Pair<t1, t2=""> { first: T1; second: T2; } |
Parámetro de tipo restringido |
(): T |
Parámetro de tipo predeterminado |
(): T |
Parámetro de tipo restringido y predeterminado |
(): T |
Tuplas genéricas |
type Arr = readonly any[]; function concat(a: N, b: V): [...N, ...V] { return [...a, ...b] } const strictResult = concat([1, 2] as const, ['3', '4'] as const); const relaxedResult = concat([1, 2], ['3', '4']); // strictResult es de tipo [1, 2, '3', '4'] // relaxedResult es de tipo (string | number)[] |
Tipos de índice, maps y condicionales | |
Consulta de tipo de índice (keyof ) |
type Point = { x: number, y: number }; let pointProp: keyof Point = 'x'; function getProp<t, k="" extends="" keyof="" t="">( val: T, propName: K ): T[K] { ... } |
Tipos map |
type Stringify = { [P in keyof T]: string; } type Partial = { [P in keyof T]?: T[P]; } |
Tipos condicionales |
type Swapper = (value: T) => T extends number ? string : number; // es equivalente a (value: number) => string // si T es un número, o (value: string) => number // si T es una cadena |
Tipos condicionales map |
interface Person { firstName: string; lastName: string; age: number; } type StringProps = { [K in keyof T]: T[K] extends string ? K : never; }; type PersonStrings = StringProps; // PersonStrings es "firstName" | "lastName" |
Otros tipos útiles | |
Partial |
Partial<{ x: number; y: number; z: number; }> // es equivalente a { x?: number; y?: number; z?: number; } |
Readonly |
Readonly<{ x: number; y: number; z: number; }> //es equivalente a { readonly x: number; readonly y: number; readonly z: number; } |
Pick |
Pick<{ x: number; y: number; z: number; }, 'x' | 'y'> // es equivalente a { x: number; y: number; } |
Record |
Record<'x' | 'y' | 'z', number> // es equivalente a { x: number; y: number; z: number; } |
Exclude |
type Excluded = Exclude; // es equivalente a number |
Extracted |
type Extracted = Extract; // es equivalente a string |
NonNullable |
type NonNull = NonNullable; // es equivalente a string | number |
ReturnType |
type ReturnValue = ReturnType<() => string>; // es equivalente a string |
InstanceType |
class Renderer() {} type Instance = InstanceType; // es equivalente a Renderer |
Tipos de Guards | |
Type predicates |
function isThing(val: unknown): val is Thing { // retorna true si val es de tipo Thing } if (isThing(value)) { // value es de tipo Thing } |
typeof |
declare value: string | number; if (typeof value === "number") { // value is of type Number } else { // value is a string } |
instanceof |
declare value: Date | Error; if (value instanceof Date) { // value is a Date } else { // value is an Error } |
in |
interface Dog { woof(): void; } interface Cat { meow(): void; } function speak(pet: Dog | Cat) { if ('woof' in pet) { pet.woof() } else { pet.meow() } } |
Assertions | |
De tipo |
let val = someValue as string; // o let val = someValue; |
const Valor inmutable |
let point = { x: 20, y: 30 } as const; // o let point = { x: 20, y: 30 }; |
Declaraciones de ámbito | |
Global |
declare const $: JQueryStatic; |
Módulo |
declare module "foo" { export class Bar { ... } } |
Módulo comodín |
declare module "text!*" { const value: string; export default value; } |
Comentarios para el compilador | |
No revisar este archivo |
// @ts-nocheck |
Verificar este archivo (JS) |
// @ts-check |
Ignora la siguiente línea |
// @ts-ignore |
Espere un error en la siguiente línea |
// @ts-expect-error |
Directivas triple slash | |
Tipos incorporados de referencia |
/// <reference lib="es2016.array.include" /> |
Referencia a otros tipos |
/// <reference path="../my_types" /> /// <reference types="jquery" /> |
AMD |
/// <amd-module name="Name" /> /// <amd-dependency path="app/foo" name="foo" /> |
Uso | |
Instalación | Para la última versión estable: $ npm install typeScript |
Ejecutar |
npx tsc |
Ejecutar con una configuración específica |
npx tsc --project configs/my_tsconfig.json |